Learn 2: Classes and Instances
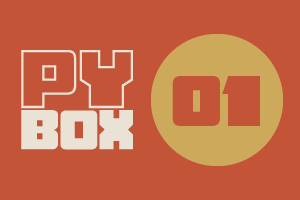
This is the first challenge within the Robotics with GoPiGo and Python Curriculum.
Your task is to program your robot to navigate an area of previously unseen terrain to reach its destination, passing through a number of waypoints.
2. Wakey, Wakey GoPiGo
Let's create an instance of our GoPiGo that we can instruct using the class library functions available to us.
Open a new notebook.
You will be presented with a single empty cell that you can type into. This is highlighted with a blue border and you will see a blinking cursor inside it. This tells you that the cell is selected.
Type the following code into the cell:
The first two lines import easygopigo3 as easy
and import time
are used to tell our program we will be using functions from the easygopigo3 and time class libraries. Since easygopigo3
is long to type we are giving it a nickname: easy.
The third line orienteer = easy.EasyGoPiGo3()
tells our program we are creating a robot orienteer instance using the nickname we gave to the easygopigo3 library. Our robot will answer to the name orienteer.
The last line orienteer.open_eyes()
tells our orienteer robot to wake up and open its eyes!
To summarize:
- easygopigo3 provides us with functions to interact with the GoPiGo3 robot.
- time provides us with time-based functions. Later on, we will use this to pause the robot.
- EasyGoPiGo3() is a constructor function for the easygopigo3 class library. This creates an instance of an easygopigo3 object.
- Let’s name our robot within the code. In programmer speak, this means that we assign the instance to a variable that we have called
orienteer
. This is our robot orienteer that we will instruct. - To instruct our robot orienteer we simply call its name and give it an instruction. In this example, we are instructing our robot orienteer to open its eyes.
NOTE
It is important to note that there is a period (.) in between the robot’s name and the open_eyes() instruction. This is important. This acts like spaces do for us humans. Imagine how difficult it would be for us to understand written text iftherewerenospaces. Be kind to your robot orienteer. Use periods. Period! 😉